Dialog
Shows a dialog that can be used to specify the script parameters.
Syntax
Dialog(doc, par1, name1, type1, par2, name2, type2, ...)
Parameters
Parameter |
Type |
Description |
---|---|---|
doc |
documentReference |
Reference to the document. Could be zero if all the type values are ‘number’ or ‘string’. |
par1 |
variable |
A variable that will be assigned a value after Dialog exits. The variable should be created before the Dialog function is called |
name1 |
string |
Prompt that will be shown in the dialog. |
type1 |
string |
Parameter type. It should be one of: ‘number’, ‘string’, ‘neuron’, ‘neuronorevent’, ‘event’, ‘interval’, ‘wave’, ‘continuous’, ‘marker’ or ‘all’. |
Return
Dialog function returns 1 if user pressed OK button or 0, if user pressed Cancel button.
Examples
Python
filefilter = "C:\\Data\\*.nex"
# show the dialog to the user
__wrapper = []
res = nex.Dialog(0., filefilter, "File Filter:", "string", __wrapper )
filefilter = __wrapper[0]
The following dialog will be shown:
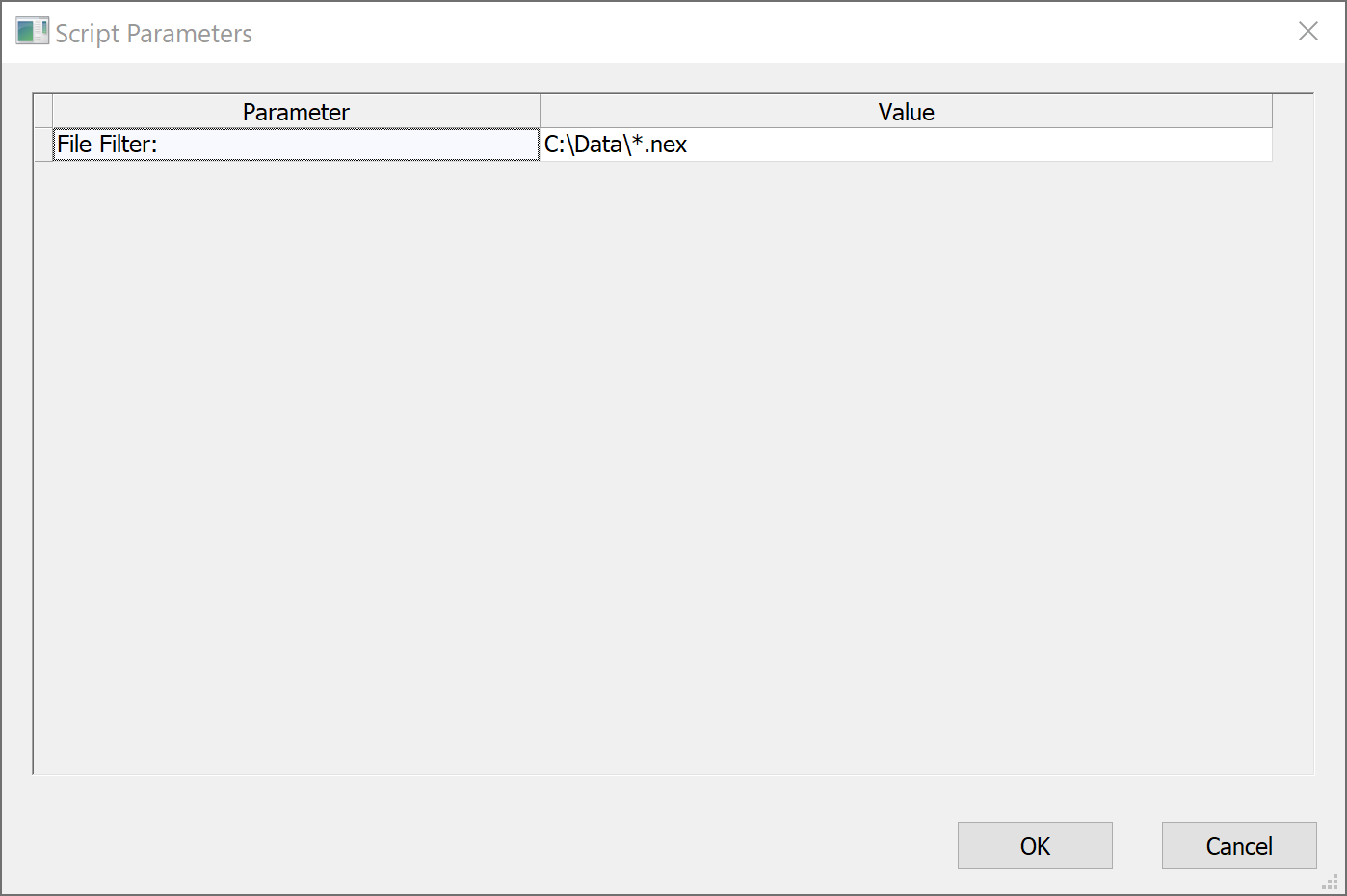
Now a user can type the new value in the File Filter edit box. If the user presses OK button, the Dialog function returns 1, otherwise, it returns 0.
The following script will allow a user to choose one of the neurons in the active document and select this neuron for analysis:
doc = GetActiveDocument()
Neuron_Number = 1
% choose a neuron
res = Dialog(doc, Neuron_Number, "Select Neuron", "neuron")
% get the neuron variable and select it
Neuron_Var = GetVar(doc, Neuron_Number, "neuron")
Select(doc, Neuron_Var)
Here is the Python equivalent:
import nex
doc = nex.GetActiveDocument()
Neuron_Number = 1
# choose a neuron
__wrapper = []
res = nex.Dialog(doc, Neuron_Number, "Select Neuron", "neuron", __wrapper )
Neuron_Number = __wrapper[0]
# get the neuron variable and select it
Neuron_Var = nex.GetVar(doc, Neuron_Number, "neuron")
nex.Select(doc, Neuron_Var)
NexScript
% create a string variable
filefilter = "C:\Data\*.nex"
% show the dialog to the user
res = Dialog(0., filefilter , "File Filter:", "string")